Introduction to SQL: Your Gateway to Mastering Database Queries
Hey, welcome to the exciting world of SQL! If you’re new to databases or just curious about how to talk to them, you’re in the right place. SQL, or Structured Query Language, is the go-to tool for working with data in databases. In this blog, we’ll walk you through what SQL is, how it works, its core components, and why it’s a must-know skill for anyone dealing with data. We’ll keep things clear, conversational, and packed with details to help you get a solid start. Let’s dive in!
What Is SQL?
SQL (pronounced “sequel” or sometimes “S-Q-L”) is a language designed to manage and manipulate data in databases. Think of it as the translator between you and a database. Want to fetch customer names, update prices, or delete old records? SQL lets you do that with simple, human-readable commands.
At its core, SQL interacts with relational databases—those organized into tables with rows and columns. It’s been around since the 1970s and remains the standard for querying databases like MySQL, PostgreSQL, Oracle, and SQL Server. Whether you’re a developer, data analyst, or business owner, SQL helps you extract meaningful insights from data.
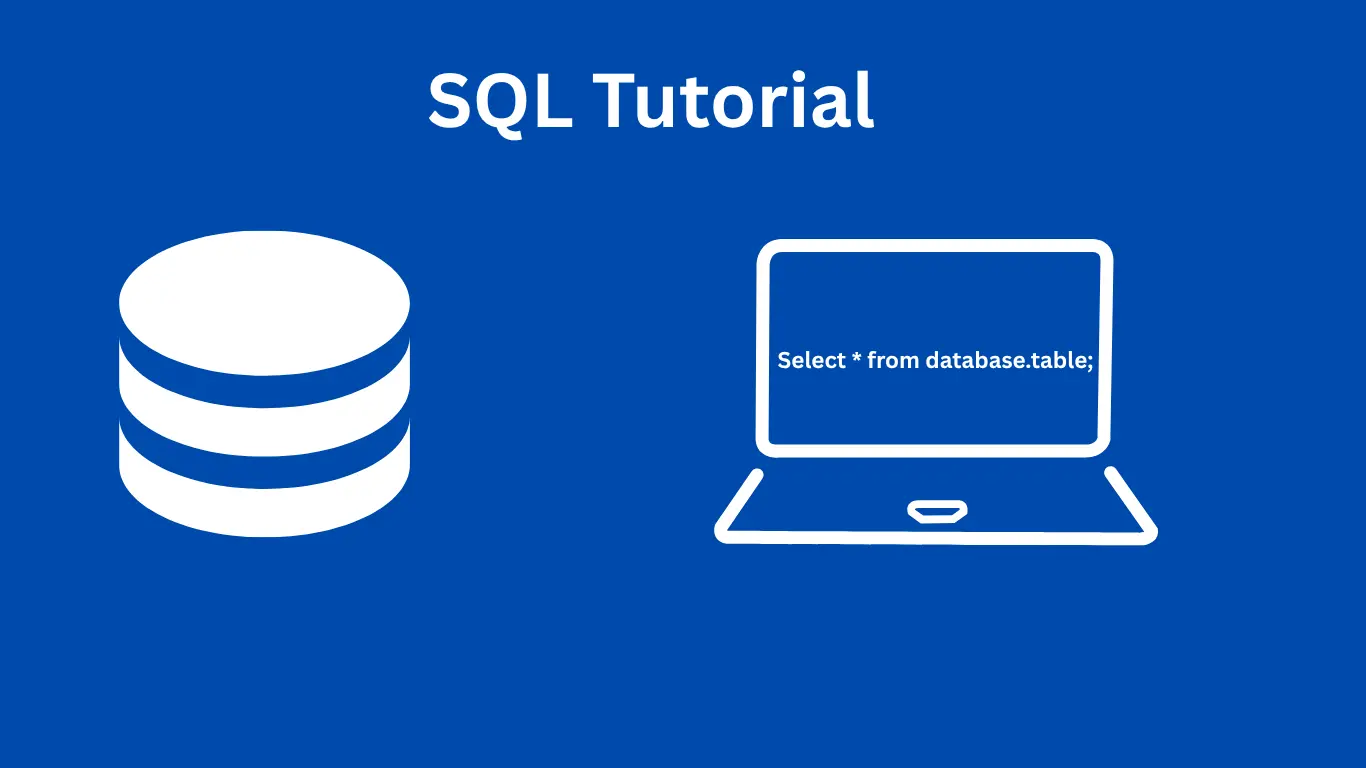
For a broader look at databases, check out Introduction to Databases. To learn more about SQL’s origins, this external history of SQL is a great read.
Why Learn SQL?
You might wonder: why bother learning SQL when there are so many tools out there? Here’s why SQL is worth your time:
- Universal Skill: SQL works across most relational databases, so learning it opens doors to many platforms.
- Data Power: It lets you ask precise questions of your data, like “Which products sold over $1,000 last week?”
- Efficiency: SQL handles large datasets quickly, unlike spreadsheets that choke on big data.
- Career Boost: From data analysts to backend developers, SQL is a top skill employers seek.
- Foundation for Advanced Tools: Many data tools (like Tableau or Python’s pandas) build on SQL concepts.
To understand how databases are structured for SQL, explore Relational Database Concepts.
Core Components of SQL
SQL is versatile, but it’s built around a few key types of commands. Let’s break them down:
Data Query Language (DQL)
DQL is all about fetching data. The star here is the SELECT statement, which retrieves data from tables. For example:
SELECT name, age FROM customers;
This pulls the name and age columns from the customers table. DQL is what you’ll use most as a beginner.
Learn more at SELECT Statement.
Data Definition Language (DDL)
DDL shapes the structure of your database. It includes commands like:
- CREATE: Builds new tables or schemas.
- ALTER: Modifies existing tables (e.g., adding a column).
- DROP: Deletes tables or databases.
Example:
CREATE TABLE products (id INT, name VARCHAR(50), price DECIMAL);
This creates a products table with columns for ID, name, and price.
Check out Creating Tables and Altering Tables.
Data Manipulation Language (DML)
DML handles the data itself. Key commands include:
- INSERT: Adds new records.
- UPDATE: Changes existing records.
- DELETE: Removes records.
Example:
INSERT INTO products (id, name, price) VALUES (1, 'Laptop', 999.99);
This adds a laptop to the products table.
Dive deeper with INSERT INTO Statement.
Data Control Language (DCL)
DCL manages access and permissions. Commands like:
- GRANT: Gives users access rights.
- REVOKE: Takes away access.
Example:
GRANT SELECT ON customers TO user1;
This lets user1 view the customers table.
For security details, see Roles and Permissions.
Transaction Control Language (TCL)
TCL ensures data integrity during changes. Commands include:
- COMMIT: Saves changes.
- ROLLBACK: Undoes changes.
- BEGIN: Starts a transaction.
Example:
BEGIN;
UPDATE products SET price = 1099.99 WHERE id = 1;
COMMIT;
This updates a price and saves the change.
Learn more at SQL Transactions and ACID.
Basic SQL Syntax
SQL is beginner-friendly because its syntax reads like English. Here’s a quick look at a SELECT query:
SELECT column1, column2
FROM table_name
WHERE condition
ORDER BY column1;
- SELECT: Chooses columns to display.
- FROM: Specifies the table.
- WHERE: Filters rows (e.g., age > 18).
- ORDER BY: Sorts results.
For a full breakdown, visit Basic SQL Syntax and WHERE Clause.
To practice formatting queries cleanly, check out SQL Query Formatting.
Setting Up Your SQL Environment
Ready to try SQL? You’ll need a database to play with. Here’s how to get started:
- Choose a DBMS: MySQL and PostgreSQL are great for beginners (and free!).
- Install It: Download and set up your DBMS. This external MySQL installation guide is super helpful.
- Get a Tool: Use a graphical tool like DBeaver or phpMyAdmin, or stick with the command line.
- Create a Database: Run CREATE DATABASE mydb; to start experimenting.
For a step-by-step setup, see Setting Up SQL Environment.
Common SQL Operations
Let’s walk through some everyday SQL tasks to give you a feel for it.
Creating a Table
Suppose you’re building a pet store database. Create a table for pets:
CREATE TABLE pets (
pet_id INT PRIMARY KEY,
name VARCHAR(50),
species VARCHAR(30),
price DECIMAL(10,2)
);
This sets up a table with a unique ID, name, species, and price. Learn about keys at Primary Key Constraint.
Adding Data
Add a few pets:
INSERT INTO pets (pet_id, name, species, price)
VALUES (1, 'Fluffy', 'Cat', 50.00),
(2, 'Rex', 'Dog', 100.00);
See INSERT INTO Statement for more.
Querying Data
Find all dogs:
SELECT name, price
FROM pets
WHERE species = 'Dog';
This returns “Rex, 100.00”. Explore filtering with WHERE Clause.
Updating Data
Raise Fluffy’s price:
UPDATE pets
SET price = 60.00
WHERE pet_id = 1;
Check out UPDATE Statement.
Deleting Data
Remove Rex:
DELETE FROM pets
WHERE pet_id = 2;
Learn more at DELETE Statement.
SQL Data Types
Every column in a table has a data type, like:
- INT: Whole numbers (e.g., 42).
- VARCHAR: Text (e.g., “Fluffy”).
- DECIMAL: Precise numbers (e.g., 50.00).
- DATE: Dates (e.g., 2025-05-25).
Choosing the right type is crucial for efficiency. For details, see Numeric Data Types and Character Data Types.
SQL in the Real World
SQL powers countless applications:
- E-Commerce: Queries product inventories or customer orders.
- Analytics: Data analysts use SQL to spot trends in sales or user behavior.
- Web Development: Backend systems fetch content for websites.
- Finance: Banks track transactions with SQL’s reliability.
For advanced use cases, explore Data Warehousing.
Challenges and Tips
SQL is powerful, but it has quirks:
- Syntax Errors: A missing semicolon can break your query. Double-check your code!
- Performance: Poorly written queries can be slow. Learn about Creating Indexes.
- Security: Bad queries can expose data. Read up on SQL Injection Prevention.
Tips:
- Practice with small datasets.
- Comment your code for clarity (SQL Comments).
- Use consistent naming (Naming Conventions).
Next Steps
You’ve got the basics of SQL! Now, try these:
- Build a Database: Create a simple one, like a book library.
- Practice Queries: Write SELECT statements with filters.
- Explore Joins: Learn to combine tables with INNER JOIN.
For a deeper dive, this external SQL tutorial is a great resource.
Wrapping Up
SQL is your key to unlocking the power of databases. With just a few commands, you can create, query, and manage data like a pro. This introduction has given you the foundation to start experimenting and building. Keep practicing, and soon you’ll be crafting complex queries with ease. Ready for more? Check out Basic SQL Syntax to refine your skills!